API(正则表达式)
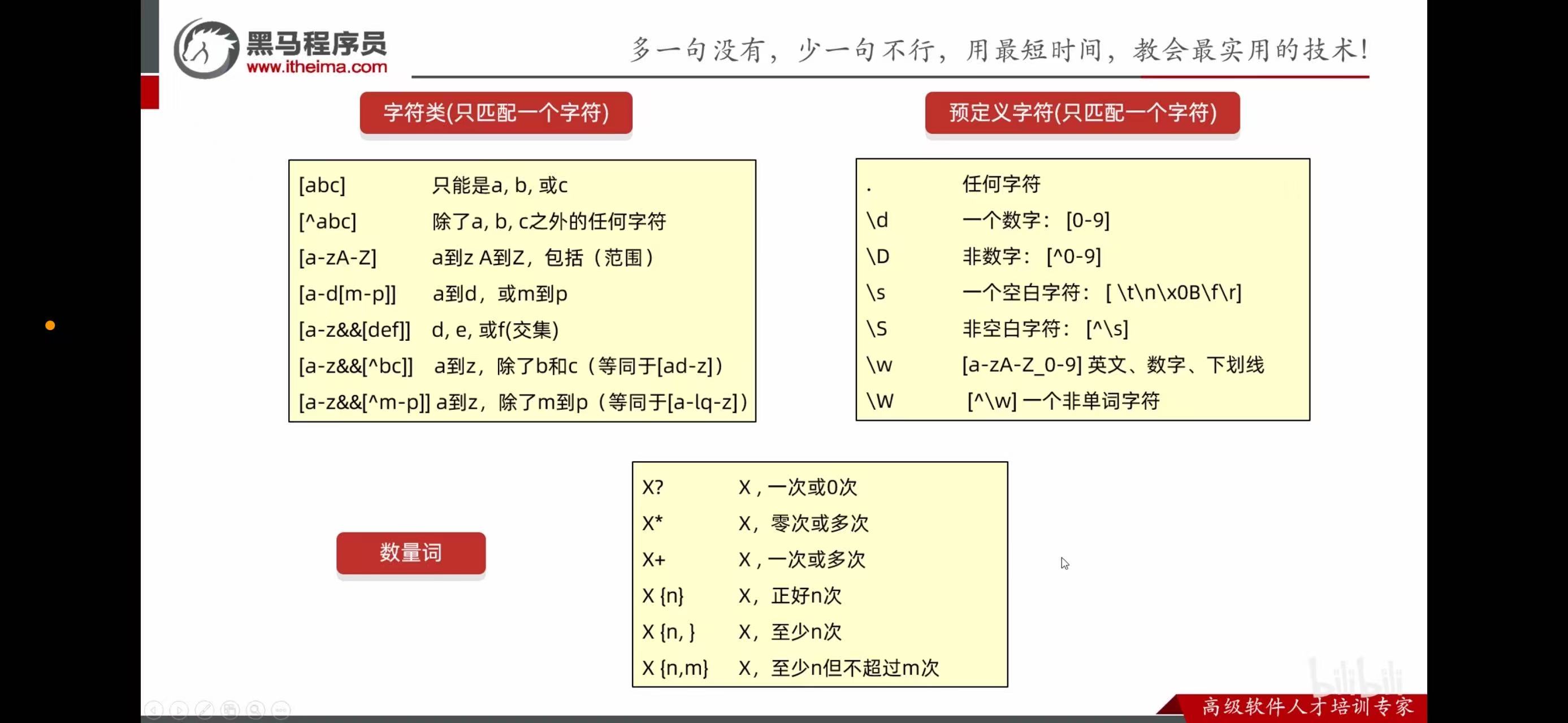
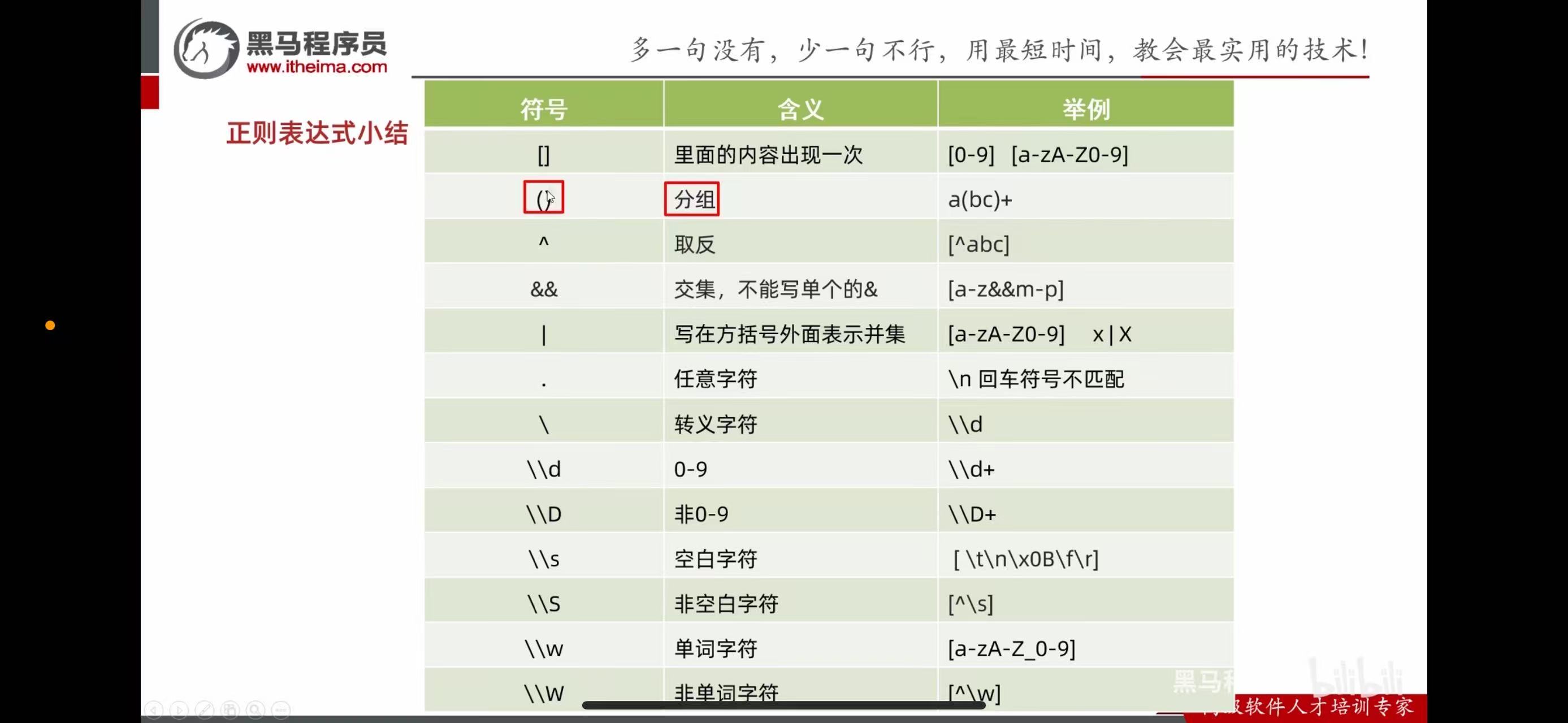
//abc中的任意一个字符
System.out.println("a".matches("[abc]"));//true(abc中的任意一个字符)
System.out.println("ab".matches("[abc]"));//false
System.out.println("z".matches("[abc]"));//false
System.out.println("a".matches("abc"));//false
//不能是abc中的任意一个字符
System.out.println("a".matches("[^abc]"));//false(^表示取反,不能是abc中的任意一个字符)
System.out.println("z".matches("[^abc]"));//true
System.out.println("zz".matches("[^abc]"));//false
System.out.println("zz".matches("[^abc][^abc]"));//true
//a-z和A-Z中的任意一个字符
System.out.println("a".matches("[a-zA-Z]"));//true
System.out.println("z".matches("[a-zA-Z]"));//true
System.out.println("aa".matches("[a-zA-Z]"));//false
System.out.println("zz".matches("[a-zA-Z][a-zA-Z]"));//true
System.out.println("0".matches("[a-zA-Z0-9]"));//true
//a-z或者A-Z中的任意一个字符(和上方类似)
System.out.println("a".matches("[a-z[A-Z]]"));//true
System.out.println("z".matches("[a-z[A-Z]]"));//true
System.out.println("aa".matches("[a-z[A-Z]]"));//false
System.out.println("zz".matches("[a-z[A-Z]]"));//false
// [a-z&&[def]] a-z和def的交集
System.out.println("a".matches("[a-z&&[def]]"));//false
System.out.println("d".matches("[a-z&&[def]]"));//true
System.out.println("f".matches("[a-z&&[def]]"));//true
System.out.println("g".matches("[a-z&&[def]]"));//false
// [a-z&&[^bc]] a-z和[^bc]的交集 即[ad-z]
System.out.println("a".matches("[a-z&&[^bc]]"));//true
System.out.println("b".matches("[a-z&&[^bc]]"));//false
System.out.println("c".matches("[a-z&&[^bc]]"));//false
// [a-z&&[^m-p]] a-z和[^m-p]的交集 即[a-lq-z]
System.out.println("a".matches("[a-z&&[^m-p]]"));//true
System.out.println("m".matches("[a-z&&[^m-p]]"));//false
System.out.println("p".matches("[a-z&&[^m-p]]"));//false
}
}
public class RegexDemo2 {
public static void main(String[] args) {
//\转义字符,改变后面字符的含义
System.out.println(“"“);//“ 打印一个双引号
//.表示任意一个字符
System.out.println("a".matches("."));//true
System.out.println("ab".matches("."));//false
System.out.println("abc".matches("..."));//true
// \d表示任意一个数字
// \\d表示转义字符,表示\d
//简单来记:两个\\表示一个\
System.out.println("a".matches("\\d"));//false
System.out.println("1".matches("\\d"));//true
System.out.println("123".matches("\\d"));//false
System.out.println("123".matches("\\d\\d\\d"));//true
// \D表示任意一个非数字
System.out.println("a".matches("\\D"));//true
System.out.println("1".matches("\\D"));//false
System.out.println("abc".matches("\\D"));//false
System.out.println("abc".matches("\\D\\D\\D"));//true
// \w表示任意一个单词字符(数字,字母,下划线)
System.out.println("a".matches("\\w"));//true
System.out.println("1".matches("\\w"));//true
System.out.println("_".matches("\\w"));//true
// \W表示任意一个非单词字符
System.out.println("a".matches("\\W"));//false
System.out.println("1".matches("\\W"));//false
System.out.println("_".matches("\\W"));//false
System.out.println("你".matches("\\W"));//true
// \s表示任意一个空白字符(空格,制表符,换行符)\t,\n,\r
System.out.println(" ".matches("\\s"));//true
System.out.println("\t".matches("\\s"));//true
System.out.println("\n".matches("\\s"));//true
System.out.println("\r".matches("\\s"));//true
// \S表示任意一个非空白字符(非空格,制表符,换行符)
System.out.println(" ".matches("\\S"));//false
System.out.println("\t".matches("\\S"));//false
System.out.println("\n".matches("\\S"));//false
System.out.println("\r".matches("\\S"));//false
System.out.println("a".matches("\\S"));//true
//以上的正则表达式都只能表示一个字符
//必须是数字 字母 下划线 至少出现4次
System.out.println("123".matches("\\w{4,}"));//false
System.out.println("1234".matches("\\w{4,}"));//true
System.out.println("12345".matches("\\w{4,5}"));//true(出现四次到五次)
//只能出现4次
System.out.println("123".matches("\\w{4}"));//false
System.out.println("1234".matches("[^\\d]{4}"));//false
System.out.println("abcd".matches("[a-z]{4}"));//true
}
}
练习
public class test2 {
public static void main(String[] args) {
String regex1 = “1[3-9]\d{9}”;//13112341234
System.out.println(“13112341234”.matches(regex1));
//?表示前面的字符出现0次或1次
String regex2="0\\d{2,3}-?[1-9]\\d{4,7}";
System.out.println("010-12345678".matches(regex2));
//邮箱号码 1234567@qq.com zhangsan@site.cn
//第一部分 @的左边 数字 字母 下划线 至少出现一次(+表示前面的字符出现1次或多次)
//第二部分 @
//第三部分 .的左边和右边
//.不能直接写要加转义字符\\.表示.
String regex3="\\w+@[\\w&&[^_]]{2,4}(\\.[a-zA-Z]{2,3}){1,3}";//()表示分组的意思
//.:在正则表达式里是元字符,匹配除换行符外的任意单个字符。
//\.:在 Java 字符串里不合法,会引发编译错误。
//\\.:在 Java 字符串里表示正则表达式的 \.,用于匹配真正的句点字符。
System.out.println("1234567@qq.com".matches(regex3));//true
System.out.println("zhangsan@site.cn".matches(regex3));//true
}
}
public class test3 {
public static void main(String[] args) {
//1.判断用户名是否合法
//要求:字母开头,长度在4~16之间,只能包含字符,数字,下划线
String regex1="\\w{4,16}";
System.out.println("zhangsan".matches(regex1));
//2.判断身份证号码是否合法
//要求:18位,不能以0开头,最后一位可以是数字或X
String regex2="[1-9]\\d{16}[xX\\d]";// (x|X|\\d)和[xX\d]是等价的
System.out.println("21043019760604644x".matches(regex2));
//(?i)表示忽略后面的所有的大小写
String regex3="(?i)\\w{4,16}";
System.out.println("Zhangsan".matches(regex3));//true
System.out.println("zhangSAN".matches(regex3));//true
//只忽略b的大小写
String regex4="a((?i)b)c";//把b括起来
System.out.println("abc".matches(regex4));//true
System.out.println("Abc".matches(regex4));//false
System.out.println("aBc".matches(regex4));//true
//3.身份证号码严格校验
//210430 1976 0604 644x
//前六位:省市县 第一位不能是0,后五位是任意数字
//中间八位:出生年月日 年前半段是18,19,20 后半段任意两数字
//月份:01-12 日:01-31
//后四位
String regex5="[1-9]\\d{5}(1[8-9]|20)\\d{2}(0[1-9]|1[0-2])(0[1-9]|[1-2]\\d|3[0-1])\\d{3}(\\d|(?i)x)";
System.out.println("21043019760604644X".matches(regex5));//true
}
}
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来源 Hexo!